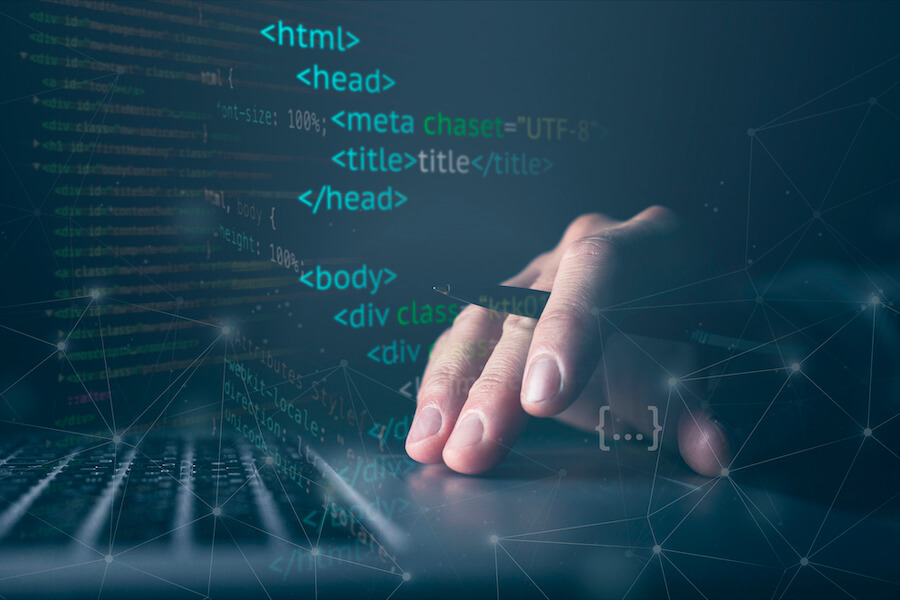
Before we delve further into the captivating realm of dynamic typing and its dual nature of advantages and pitfalls, it's crucial to lay down a strong foundation by discussing the various types that inhabit the vast universe of JavaScript. These types are the very essence of the data you store within variables, forming the building blocks upon which the language operates.
However, before we dive into the specifics, let's take a moment to decipher the term "primitive type." Think of a primitive type as the elemental form of data, representing a solitary value—something that exists in isolation, devoid of complex structures. Unlike objects, which bundle data and properties together, primitive types encapsulate individual values.
In the vibrant world of JavaScript, there exist six fundamental primitive types that underpin the language. Let's take a closer look at each one
Primitive Types
Undefined: The Absence of Definition
Picture a vast, empty space—a canvas yet to be painted. This is the essence of the undefined
primitive type. When a variable is declared but not assigned a value, the JavaScript engine automatically designates it as undefined
. It symbolizes an absence of existence, a placeholder awaiting significance. It's important to note that you shouldn't explicitly set a variable to undefined
; let it remain as a flag indicating uninitialized variables.
Null: The Explicit Emptiness
Parallel to undefined
, the null
primitive type also signifies nonexistence. However, unlike its counterpart, null
is a deliberate choice—a conscious assignment of emptiness. By setting a variable to null
, you communicate that it possesses no meaningful data. It's the appropriate tool to signify that the variable deliberately holds no value.
Boolean: The Realm of True and False
Step into the realm of binary decisions, where choices are either black or white—welcome to the domain of the Boolean
primitive type. This foundational data type embraces simplicity, offering two options: true
or false
. It forms the bedrock of logical operations, serving as the compass for conditional statements and branching within your code.
Number: Mathematical Building Blocks
JavaScript's numeric prowess unfolds through the Number
primitive type. Here, numbers are your mathematical companions, aiding in calculations and operations. However, it's essential to grasp that JavaScript's numeric type is inherently a floating-point number. Even integers bear decimal potential, a characteristic that sometimes introduces intriguing mathematical nuances.
String: The Tapestry of Textual Data
Strings, those sequences of characters that craft language and communication, are another pivotal component of JavaScript. Whether encapsulated within single quotes or double quotes, strings offer the conduit to represent textual data. They bring life to variable names, function labels, and user input.
Symbol: The Unique Identifier (ES6)
Introducing the newest member of the primitive lineup—the symbol
type. While we won't delve deep due to its ES6 origins and limited browser support, its existence is noteworthy. Imagine symbols as distinct, immutable values used as object property keys to thwart potential naming conflicts.
Putting It Into Action: Examples
Undefined and Null
let a; // 'a' is undefined
let b = null; // 'b' is explicitly set to null
Boolean
const isOpen = true;
const isLoggedOut = false;
Number
const age = 27;
const pi = 3.14159;
String
const greeting = "Hello, world!";
const username = 'Alice';
Symbol: The Unique Identifier (ES6)
// Create unique symbols for cache keys
const cacheKeyA = Symbol('cacheKeyA');
const cacheKeyB = Symbol('cacheKeyB');
// Create the cache object
const cache = {};
// Store values using symbols as keys
cache[cacheKeyA] = 'Cached value for Key A';
cache[cacheKeyB] = 'Cached value for Key B';
// Retrieve values using symbols as keys
console.log(cache[cacheKeyA]); // Output: Cached value for Key A
console.log(cache[cacheKeyB]); // Output: Cached value for Key B
In this example, we create two distinct symbols, cacheKeyA
and cacheKeyB
, which serve as unique identifiers for our cache keys. By utilizing symbols, we ensure that the properties used for caching won't inadvertently conflict with any other properties, enhancing the robustness of our library.
Conclusion
While delving into the intricacies of primitive types may not be the most exhilarating start to our JavaScript journey, it's a crucial foundation that paves the way for understanding more complex concepts ahead. The six primitive types—undefined
, null
, Boolean
, number
, string
, and symbol
—lay the groundwork for JavaScript's dynamic typing, allowing the language to adapt to the data types it encounters. As we move forward, armed with this knowledge, we're poised to explore the dynamic nature of JavaScript's type system and how it shapes our coding experiences.
Source
The content of this blog post is based on JavaScript concepts and principles. Additional insights and examples have been curated from reputable JavaScript resources, including:
- MDN Web Docs: https://developer.mozilla.org/en-US/docs/Web/JavaScript
- "JavaScript: The Definitive Guide" by David Flanagan