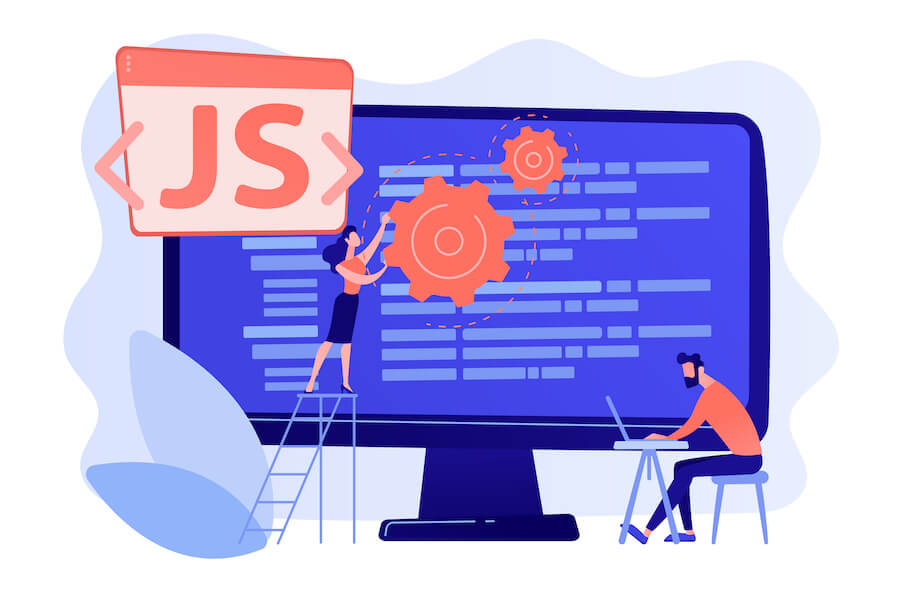
In the programming world, you might encounter unfamiliar symbols or operators that leave you scratching your head.
One such symbol that might have caught your eye is the double question mark (??). You're in the right place if you’re wondering what this mysterious operator does and how it can simplify your code.
In this article, we will delve into the world of the nullish coalescing operator and explore its significance in JavaScript and TypeScript.
What is the Nullish Coalescing Operator (??)?
The double question marks (??) are formally known as the nullish coalescing operators. These operators provide a convenient way to handle default values in your code when dealing with variables that might be null or undefined.
In simple terms, the nullish coalescing operator allows you to set a default value on the right side of the operator if the value on the left side is null or undefined.
Let’s illustrate this concept with an example:
let random;
const color = random ?? 'red';
In this code snippet, we have two variables: random and color. Since random is undefined, the color variable is assigned the default value of “red.”
Difference Between Logical OR (||) and Nullish Coalescing Operator (??)
Before diving deeper into the nullish coalescing operator, it’s essential to understand how it differs from another commonly used operator in JavaScript: the logical OR (||).
Consider the following code using the logical OR operator:
let random;
const color = random || 'red';
In this scenario, if random is falsy (i.e., evaluates to false when converted to a boolean), the color variable will be assigned the default value of “red.”
Here’s where the key difference lies:
The logical OR operator uses the default value if the initial expression is considered falsy. Common falsy values in JavaScript include false, 0, null, and undefined. These values, when converted to a boolean, always result in false.
console.log(Boolean(false)); // false
console.log(Boolean(0)); // false
console.log(Boolean(null)); // false
console.log(Boolean(undefined)); // false
In contrast, the nullish coalescing operator (??) uses the default value only when the initial expression is specifically null or undefined. Any other falsy values will not trigger the use of the default value.
console.log(0 ?? 'default'); // 0
console.log(false ?? 'default'); // false
console.log(null ?? 'default'); // default
console.log(undefined ?? 'default'); // default
The nullish coalescing operator was introduced to address situations where developers using the logical OR operator unintentionally triggered the default value due to a lack of clarity regarding all the falsy values in JavaScript.
Conclusion
In summary, the nullish coalescing operator (??) is a valuable addition to JavaScript and TypeScript that simplifies the handling of default values in cases where variables may be null or undefined. Its distinction from the logical OR operator (||) lies in its precise treatment of null and undefined values, ensuring that default values are used only when necessary.