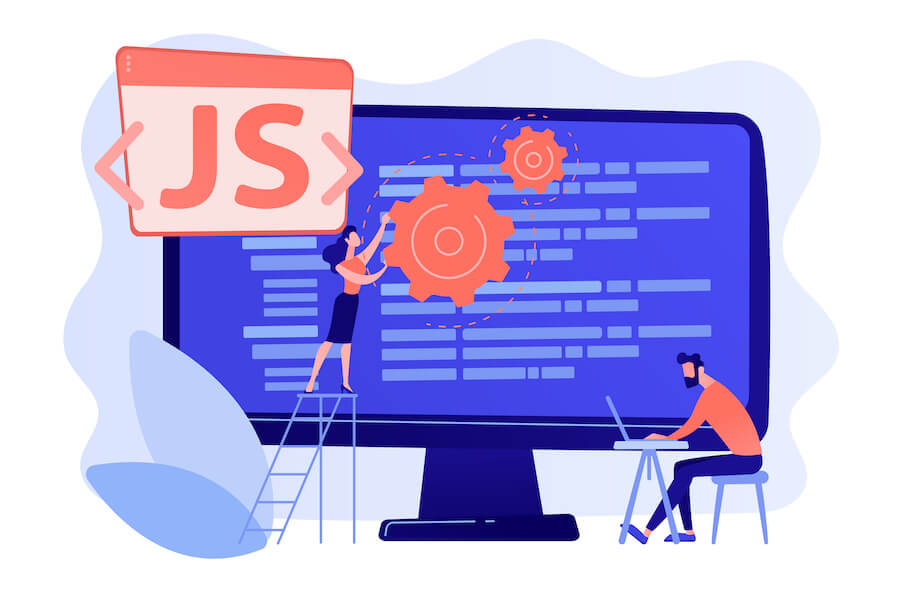
Have you ever seen !! in someone’s code and had no idea what it does? In short, The double exclamation mark (!!) is a JavaScript operator that instantly transforms any value into its corresponding boolean form. Known as the “double bang” or “not not” operator, it’s a quick and easy way to convert a value for use in conditional statements. This operator is also handy for negating boolean values and checking for the existence of variables or object properties. A must-have tool for any web developer, the double exclamation mark allows you to check the truthiness or falseness of a value and make decisions based on the results with ease. Same as Boolean(). If you are still not sure then let me explain it a bit more in detail and tell you why you WILL find it useful!
Boolean Conversion
How the double exclamation mark convert to a boolean
The double exclamation mark operates by taking the value on its right and converting it to a boolean. It does this by using the negation operator (!) to invert the value, then inverting it again to reveal its true boolean form. Imagine having the ability to flip a switch, turning a value’s boolean form from true to false, and back again. For example, if the value is true, it becomes false, and vice versa. Then it applies the negation operator again, which inverts the boolean value back to its original value. This process of applying the negation operator twice effectively converts the value to its corresponding boolean value.
“Truthy” and “falsy” in JavaScript
There exist certain values that will be evaluated as true in a boolean context, such as in a conditional statement, these are known as “truthy” values. Examples of these powerful values include non-empty strings, non-zero numbers, objects, and arrays. On the other hand, some values basically lack any value, and when used in a boolean context, they are evaluated as false, these are known as “falsy” values. Examples of these values include empty strings, the number 0, null, undefined, and NaN.
The double exclamation mark holds the key to unlocking the truth behind a value, revealing its true nature as a truthy or falsy value. With its power, a truthy value will return true and a falsy value will return false
Both methods possess the power to uncover the truth behind a value, but the double exclamation mark is a master of simplicity and ease of reading. However, the Boolean() function can be useful in situations where an explicit indication of a value’s conversion to a boolean is required, while the double exclamation mark may be less clear in this regard. It can be considered as a shorthand for the Boolean() function, but be aware that it can exhibit unique behavior in certain edge cases.
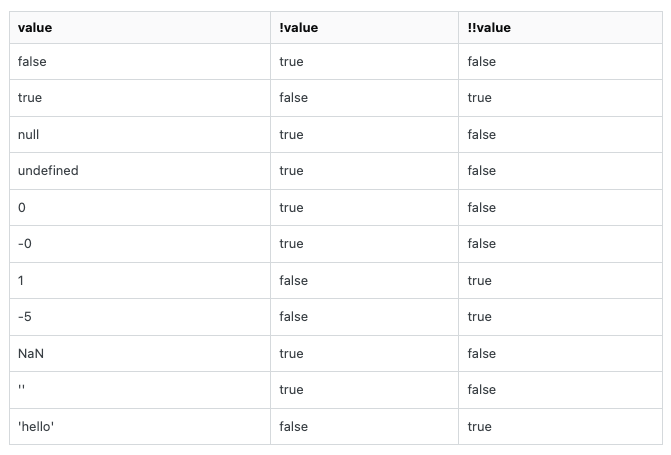
Use cases
One common use is negating a boolean value. By using the double exclamation mark in front of a boolean value, you can invert its value, effectively negating it. For example, !!true returns false, and !!false returns true. This can be useful in situations where you need to check for the opposite of a boolean value. And yes you can use single as well.
Another use for the double exclamation mark is in situations where you need to check for the existence of an object or array before acting. For example, if you want to check if an object has a certain property before performing an action, you can use the double exclamation mark to check for the truthiness of the object property, like this:
if (!!object.property) {
// perform action
}
Additionally, you can use it to check the truthiness of a variable before performing an action, like this:
const variable;
if(!!variable) {
// perform action
}
This can be useful in situations where you want to check if a variable has been defined before using it.
It’s also common to use it in combination with other operators to test the results of some conditions, like this:
const variable = 0;
if (!!variable && variable !== 0) {
//perform action
}
Conclusion
In summary, the double exclamation mark is a powerful JavaScript operator that can be used to convert values to booleans, check for truthy or falsy values, negate boolean values, check for the existence of object properties or variables, and more. Its versatility makes it a worth-knowing tool for any web dev.
While the double exclamation mark has many benefits such as its conciseness, ease of reading, and versatility, it’s important to be aware of its potential drawbacks such as its less clear usage in certain situations and the potential for unexpected results or errors when used with undefined or null values. Also, the majority of juniors won’t be able to tell what it is.