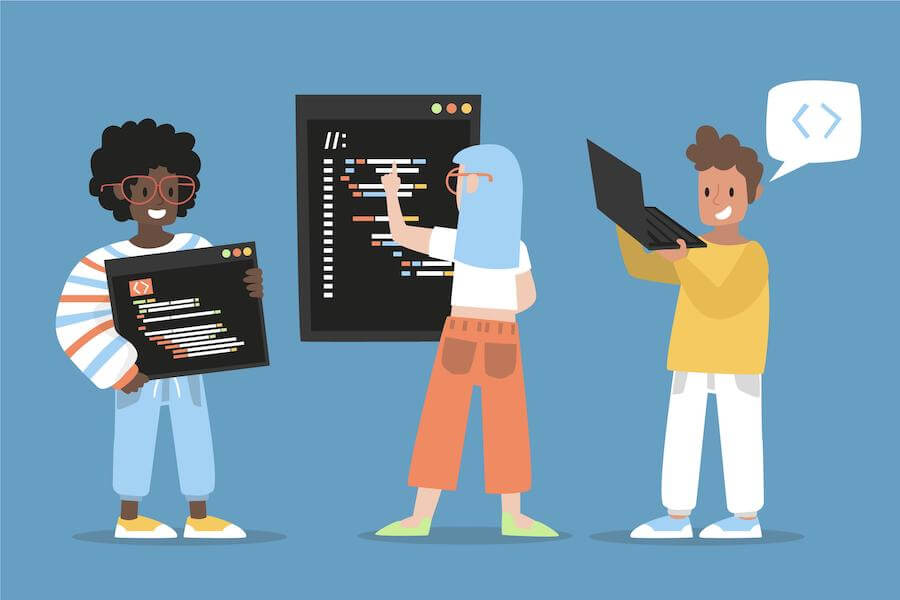
JavaScript console tips & tricks
The JavaScript console is an indispensable tool for developers seeking to understand their code's behavior and identify errors. While the console's primary function is straightforward – to output messages – it offers a treasure trove of features that can greatly enhance your debugging process. In this article, we'll uncover some valuable tips and tricks to help you maximize your use of the JavaScript console.
Computed property names
ES6 computed property names are particularly useful, as they can help you identify logged variables by adding a pair of curly braces around them.
const x = 1, y = 2, z = 3;
console.log({x, y, z}); // {x: 1, y: 2, z: 3}
console.trace()
console.trace()
works the exact same as console.log()
but it also outputs the entire stack trace so you know exactly what's going on.
const outer = () => {
const inner = () => console.trace('Hello');
inner();
};
outer();
/*
Hello
inner @ VM207:3
outer @ VM207:5
(anonymous) @ VM228:1
*/
console.assert()
console.assert()
provides a handy way to only log something as an error when an assertion fails (i.e. when the first argument is false
), otherwise, skip the log entirely
const value = 10;
console.assert(value === 10, 'Value is not 10!'); // Nothing is logged
console.assert(value === 20, 'Value is not 20!'); // Logs "Value is not 20!"
Log with Contextual Information
Adding context to your logs can provide valuable insights. You can include the function name or a relevant identifier to understand better where the log is coming from.
function processData(data) {
console.log('Processing data:', data);
}
processData({ id: 1, name: 'John' });
Dynamic Logging with Template Literals
Utilize template literals to dynamically construct log messages, combining strings and variable values.
const username = 'Alice';
console.log(`User ${username} logged in.`);
Tabular Data Display
When dealing with arrays or objects, you can use console.table
to display tabular data, making it easier to visualize structured information.
const users = [
{ id: 1, name: 'Alice', age: 25 },
{ id: 2, name: 'Bob', age: 30 },
];
console.table(users);
Logging Errors and Exceptions
console.error
is specifically designed for logging errors and exceptions, making them distinct from regular logs.
try {
throw new Error('Something went wrong!');
} catch (error) {
console.error('An error occurred:', error);
}
Counting Occurrences
You can use console.count
to keep track of how many times a particular log statement is executed.
function performAction(action) {
console.count(`Action: ${action}`);
// Code to perform the action
}
performAction('Click');
performAction('Click');
performAction('Hover');
Grouping with Collapsed Logs
Organize related logs within a group, and collapse or expand the group as needed for better readability.
console.groupCollapsed('Processing User Data');
console.log('Fetching user...');
console.log('Processing user...');
console.groupEnd();
Highlighting Important Logs
Use CSS styling to make critical logs stand out, ensuring they catch your attention.
console.log('%cImportant Message', 'color: red; font-weight: bold;');
Custom Objects Inspection
For custom objects, you can implement the .inspect()
method to control how they're displayed in the console.
const customObject = {
name: 'Custom Object',
inspect() {
return `Name: ${this.name}`;
},
};
console.log(customObject);
Conditional Logging
You can conditionally log messages based on certain conditions, which can be particularly helpful for debugging specific scenarios.
const debugMode = true;
if (debugMode) {
console.log('Debug information...');
}
Clearing the Console
When your console becomes cluttered, use console.clear()
to wipe the slate clean.
console.clear();
Logging Objects and Circular References
When logging objects with circular references, use console.dir
for more comprehensive output.
const circularObj = { prop: null };
circularObj.prop = circularObj;
console.dir(circularObj);
Timing Code Execution
Measure how long it takes for a portion of your code to run using console.time
and console.timeEnd
.
console.time('Timer');
// Code you want to measure
console.timeEnd('Timer'); // Output: Timer: 42.123ms
Conclusion
The console.log
function is far more than just a simple tool for printing messages to the console. Armed with these advanced tips and tricks, you can wield it as a versatile debugging instrument. From logging with context to dynamically constructing messages, formatting output, and more, these techniques will empower you to tackle complex issues with precision. As you incorporate these tricks into your debugging arsenal, you'll not only gain a deeper understanding of your code but also become a more efficient and effective JavaScript developer.