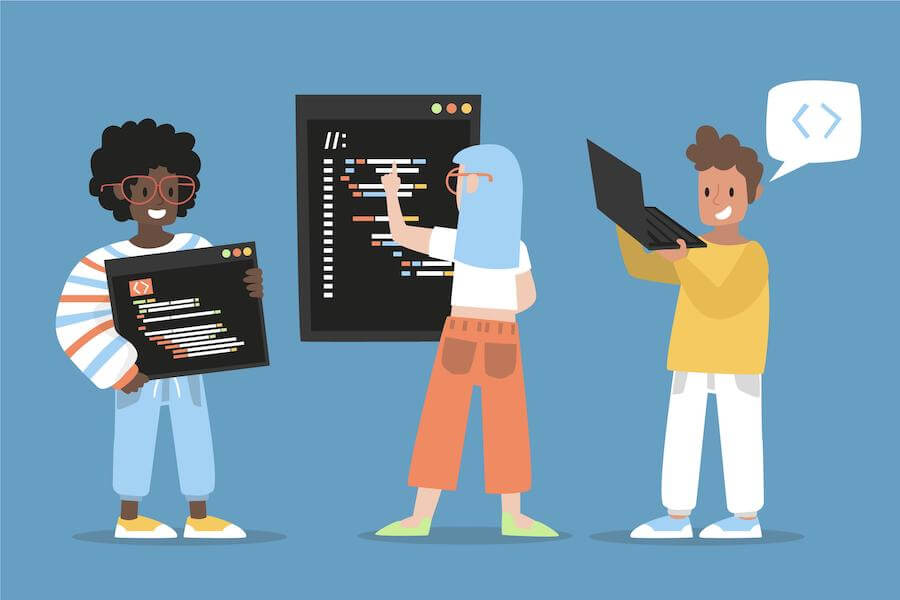
The JavaScript programming language is jam-packed with features, functions, methods, objects, and more, which makes programming much easier for all developers. In this post, we will discuss the benefits of the JavaScript set object and how to use it. We will also cover how it works and how to use it in your programs or software. Furthermore, we will look at the methods that manipulate and modify the set object during development.
What is a set in JavaScript?
JavaScript is a powerful programming language that offers a variety of ways to manage data and collections of data. One of those ways is the JavaScript object known as sets, which is used specifically on data collections. If you aren't familiar with JavaScript you should check out our post on the basics of JavaScript.
Like any other object in JavaScript, there are rules about how sets function and how they are used. Sets are data objects that hold a collection of unique values.
Sets can hold data of any type, but may not contain duplicate items. In the case of adding duplicate and equal items, only the first instance will be saved to the set.
Set Object Explained
The Set
object allows you to store a collection of elements, just like an Array. The differences between a Set
and an array are:
- A
Set
requires all elements to be unique - A
Set
has fewer methods for data manipulation
How to Create a Set Object
To create a new Set
object, you need to call the Set()
constructor as follows:
const mySet = new Set();
The code above will create a new empty set.
Set Object Methods and Properties
A Set
object has the following methods and properties:
add(value)
– Adds a value to a Sethas(value)
– Checks if a Set contains a specific valuedelete(value)
– Removes a specific value from a Setclear()
– Removes all items from a Setkeys()
– Returns all values in a Setvalues()
– Returns all values in a Setentries()
– Returns all values in a Set as[value, value]
arraysize
– Returns the number of items in Set
Note that the keys()
and values()
methods in a Set object return the same output.
There's also the entries()
method which returns an array as follows:
const mySet = new Set(['Xuho', 'Nguyen Hoa', 'Hoanx']);
console.log(mySet.entries());
Output:
Notice how the values are repeated once in each array above. The entries()
method is created to make Set
similar to the Map
object, but you probably don't need it.
There are extra methods that you can use to interact with another Set
object. We'll discuss them in the next section.
To add an element to the Set object, you can use the add method:
const mySet = new Set();
mySet.add(1);
mySet.add(2);
mySet.add(3);
console.log(mySet); // [1, 2, 3]
To get all values stored in a Set
, call the values()
method:
mySet.values(); // [Set Iterator] { 'Xuho', 'Nguyen Hoa', 'Hoanx' }
To check if the Set
has a specific value, use the has()
method:
mySet.has('Xuho'); // true
mySet.has('Xuho95'); // false
To remove a single value, call the delete()
method. To remove all values, use the clear()
method:
mySet.delete('Xuho');
mySet.clear();
Set Composition Methods
Aside from the regular methods above, Set
also has composition methods that you can use to perform various set theory operations such as difference, union, and intersection.
The following table is from MDN Set documentation:
For example, you can get a set containing the differences between two other sets as follows:
const setA = new Set([1, 2, 3, 4, 5]);
const setB = new Set([4, 5, 6, 7, 8]);
const diffsA = setA.difference(setB); // Set(3) {1, 2, 3}
const diffsB = setB.difference(setA); // Set(3) {6, 7, 8}
Here, the setA.difference(setB)
returns a Set
containing values unique to the setA
object.
The opposite values are returned when you run setB.difference(setA)
method.
Note that these methods are new additions to the JavaScript standard, and as of this writing, only Safari 17 and Chrome 122 support these methods.
Most likely, these methods will be included in Node.js soon.
Iterate Over a Set Object
To iterate over a Set
object, you can use either the forEach()
method or the for .. of
loop:
const mySet = new Set(['Xuho', 'Nguyen Hoa', 'Hoanx']);
// iterate using the forEach() method
mySet.forEach(value => {
console.log(value);
});
// or using the for .. of loop
for (const value of mySet) {
console.log(value);
}
Output:
Xuho
Nguyen Hoa
Hoanx
When to Use the Set Object
You can think of the Set
object as the alternative version of the regular Array.
Because a Set
object ignores duplicate values, you can use this object to remove duplicate items in array Javascript, then turn the Set
object back to an Array:
const myArray = [1, 1, 2, 2, 3, 3];
const uniqueArray = [...new Set(myArray)];
console.log(uniqueArray); // [ 1, 2, 3 ]
Or you can use
const myArray = [1, 1, 2, 2, 3, 3];
const uniqueArray = Array.from(new Set(myArray));
console.log(uniqueArray); // [ 1, 2, 3 ]
Another reason you may want to use a Set
is when you need to compose multiple set objects using the composition methods, such as union()
and difference()
. These methods are not available in an Array.
Conclusion
In this article, you've learned the Set object work and when to use it in your code.
Happy coding 😉