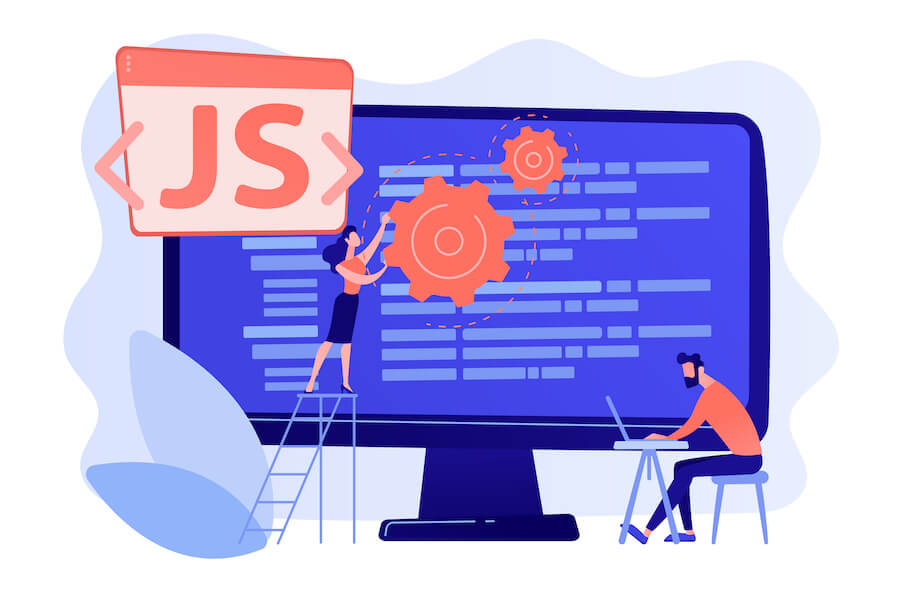
JavaScript is a powerful and versatile programming language that allows developers to build dynamic and interactive web applications. To fully understand JavaScript's behavior, it's essential to grasp the concept of execution context, creation, and hoisting. In this article, we'll delve into these topics and explore how they affect the execution of JavaScript code.
Execution Context and Wrapping Code
When JavaScript code is executed, the JavaScript engine wraps the code in an execution context. This execution context contains information about the code being executed, such as variables, functions, and the scope chain. Understanding execution context is crucial because it helps us comprehend how JavaScript behaves during runtime.
Hoisting: A Surprising Phenomenon
One of the peculiar aspects of JavaScript is hoisting, which often surprises and confuses developers. In most programming languages, executing code that references variables or functions before they are declared would result in an error. However, JavaScript behaves differently.
Consider the following code snippet:
console.log(a);
b();
function b() {
console.log("Called b");
}
var a = "Hello World";
console.log(a);
At first glance, you might expect an error because the function b
is invoked before it's declared. However, in JavaScript, hoisting allows the code to run without errors. When you execute this code, you'll see the following output:
undefined
Called b
Hello World
Even though the function b
is declared below its invocation, JavaScript doesn't throw an error. Instead, it assigns the value undefined
to a
before it's explicitly set to "Hello World"
. This behavior can be perplexing, but it's crucial to understand how JavaScript's execution context is created to make sense of it.
Let's see what exactly Javascript do
var a;
console.log(a);
function b() {
console.log("Called b");
}
b();
a = "Hello World";
console.log(a);
Creation Phase and Hoisting
To comprehend why hoisting occurs, we need to delve into the process of creating an execution context in JavaScript. The creation of an execution context happens in two distinct phases: the creation phase and the execution phase.
During the creation phase, the JavaScript engine sets up the execution context by creating the global object and the this
keyword. The global object is a reference to the global scope, and it plays a vital role in the execution of code within that scope.
In the creation phase, the JavaScript engine also hoists variables and functions. Hoisting doesn't physically move the code to the top of the page; instead, it allocates memory space for variables and functions before executing the code.
In our previous example, during the creation phase, the JavaScript engine sets aside memory space for a
and b
. However, it doesn't assign the value of a
until the execution phase. That's why console.log(a)
outputs undefined
.
Hoisting and Variables
Hoisting treats variables and functions differently. While functions are entirely placed into memory during the creation phase, variables are assigned a placeholder value called undefined
. This placeholder indicates that the variable exists but doesn't have a defined value yet.
It's essential to note that relying on hoisting can lead to confusion and potential bugs. Depending on hoisting can result in unexpected values, as demonstrated when accessing a
before assigning its value explicitly. Therefore, it's considered best practice to always declare and initialize variables before using them.
Execution Phase and Code Execution
After the creation phase, the execution phase begins. In this phase, the JavaScript engine executes the code line by line, assigning values to variables and executing function code. During the execution phase, the code has access to the variables and functions that were hoisted in the creation phase.
To ensure predictable behavior and avoid potential pitfalls, it's crucial to understand that hoisting doesn't change the order of code execution. It's simply a mechanism that allows the JavaScript engine to allocate memory for variables and functions before executing the code.
Conclusion
JavaScript's execution context, creation phase, and hoisting are fundamental concepts for understanding the behavior of JavaScript code. Hoisting can be surprising, but once you grasp the underlying mechanisms, you'll be able to navigate JavaScript's intricacies more effectively.
Remember that hoisting applies differently to variables and functions. While functions are entirely placed into memory during the creation phase, variables are assigned the placeholder value undefined
until their actual value is assigned during the execution phase.
By understanding how the execution context is created and the behavior of hoisting, you can write cleaner, more predictable JavaScript code.