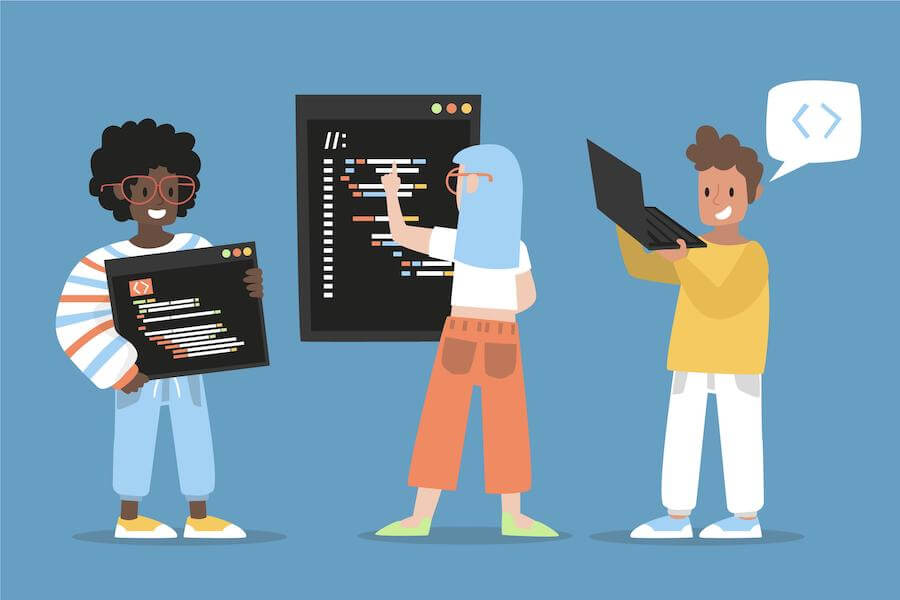
In the fast-paced world of web development, writing clean and maintainable code is crucial for the long-term success of any project. JavaScript, being one of the most popular programming languages for building interactive web applications, requires adherence to coding conventions to ensure consistency, readability, and collaboration among developers. In this blog post, we will explore some of the best practices for JavaScript coding conventions to help you write better code and make your projects more manageable.
Consistent Indentation and Formatting
Consistency in indentation and code formatting enhances code readability. Use either tabs or spaces for indentation, and choose a fixed number of spaces (commonly 2 or 4). Automated tools like ESLint or Prettier can help enforce these rules across your codebase.
// Bad indentation and formatting
function exampleFunction(){
let x=5;
if(x>3){
x= x+1;
console.log(x);
}
}
// Good indentation and formatting
function exampleFunction() {
let x = 5;
if (x > 3) {
x = x + 1;
console.log(x);
}
}
Meaningful Naming Conventions
Choose descriptive and meaningful names for variables, functions, and classes. Use camelCase for variable and function names, and PascalCase for class names. Avoid abbreviations or overly cryptic names, as they can make the code difficult to understand.
// Bad variable name
const a = 10;
// Good variable name
const numberOfStudents = 10;
Avoid Global Variables
Global variables can lead to unexpected side effects and make it harder to understand how data flows through your application. Encapsulate variables within functions or modules to limit their scope.
// Bad: Global variable
var globalVariable = 42;
function addOneToGlobal() {
globalVariable += 1;
}
// Good: Encapsulate variable within a function
function addOneToNumber(number) {
return number + 1;
}
let number = 42;
number = addOneToNumber(number);
Use Constants for Fixed Values
When you have fixed values that don't change during the program's execution, use constants (const) to represent them. This practice enhances code readability and reduces the chance of accidental value modification.
// Bad: Using magic numbers
function calculateCircleArea(radius) {
return 3.14 * radius * radius;
}
// Good: Using constants
const PI = 3.14;
function calculateCircleArea(radius) {
return PI * radius * radius;
}
Always Declare Variables
JavaScript allows declaring variables without using the "var," "let," or "const" keywords, but doing so can lead to accidental global variable creation or scoping issues. Always declare your variables explicitly using "var," "let," or "const" to prevent unexpected behavior.
// Bad: Undeclared variable
function badExample() {
x = 5;
console.log(x);
}
// Good: Declare the variable
function goodExample() {
let x = 5;
console.log(x);
}
Consistent Bracing Style
Choose a bracing style (e.g., Allman, K&R, or Stroustrup) and stick to it throughout your codebase. Consistent bracing enhances readability and reduces confusion.
// Bad bracing style
function badStyle() {
if (condition)
{
// code
}
else
{
// code
}
}
// Good bracing style
function goodStyle() {
if (condition) {
// code
} else {
// code
}
}
Avoid Nested Callbacks
Avoid deeply nested callbacks and consider using modern features like Promises or async/await to write more elegant and maintainable asynchronous code.
// Bad: Deeply nested callbacks
getData(function (data) {
getMoreData(data, function (moreData) {
processData(moreData, function (result) {
displayResult(result);
});
});
});
// Good: Using Promises
getData()
.then(getMoreData)
.then(processData)
.then(displayResult)
.catch(handleError);
Comment Your Code Wisely
Use comments to explain complex logic, algorithms, or any non-obvious code. However, avoid unnecessary comments that merely restate what the code already shows.
// Bad: Unnecessary comment
function add(a, b) {
// This function adds two numbers
return a + b;
}
// Good: Commenting complex logic
function calculateTax(income) {
// Calculate tax based on income brackets and deductions
// ...
return taxAmount;
}
Modularize Your Code
Break down your code into smaller, manageable modules, each responsible for a specific task. This helps with code organization, and maintainability, and encourages code reuse
// Bad: A large monolithic function
function processUserData(userData) {
// code
}
function handleUserData(userData) {
// code
}
function mainFunction(userData) {
processUserData(userData);
handleUserData(userData);
}
// Good: Modularized functions
function processUserData(userData) {
// code
}
function handleUserData(userData) {
// code
}
function mainFunction(userData) {
processUserData(userData);
handleUserData(userData);
}
Use Arrow Functions Sparingly
While arrow functions can make code concise and enhance readability, use them judiciously. For complex functions or methods, regular function expressions may be more explicit and easier to follow.
// Bad: Complex logic in an arrow function
const addNumbers = (a, b) => a + b;
// Good: Regular function expression for complex logic
function addNumbers(a, b) {
// complex logic here
return a + b;
}
Handle Errors Gracefully
Always implement error handling in your code to prevent unexpected crashes. Use try-catch blocks when appropriate and provide meaningful error messages for debugging.
// Bad: No error handling
function badExample() {
const result = someFunction();
// code that depends on result
}
// Good: Error handling with try-catch
function goodExample() {
try {
const result = someFunction();
// code that depends on result
} catch (error) {
// handle the error gracefully
}
}
Conclusion
By following these JavaScript coding conventions' best practices, you can write cleaner, more maintainable code, making your projects easier to collaborate on and maintain in the long run. Consistency, readability, and a focus on best practices will not only benefit you as a developer but also contribute to the success of your team's efforts and the overall quality of the software you build. Happy coding!