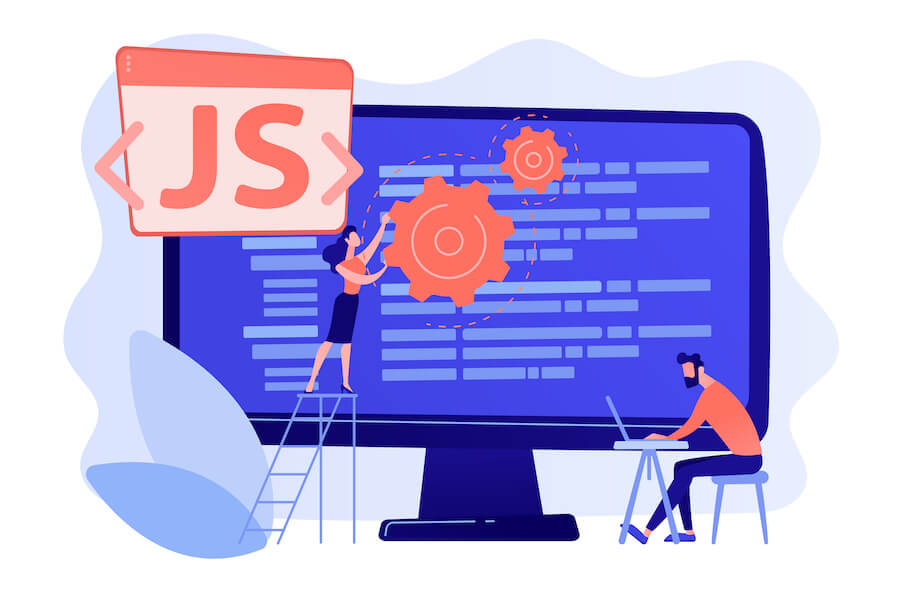
Working with dates in JavaScript can be tricky, as it requires dealing with various date formats and time zones. JavaScript does offer built-in methods for handling dates. Still, in many cases, you might find it beneficial to use a library to ensure consistent and accurate date handling, regardless of the source or display format of the date.
When working with dates, one common task is formatting them into a specific format. The format can involve different components like day, month, year, and separators like slashes or dashes. Some of the widely used date formats include:
- ISO 8601: YYYY-MM-DDTHH:mm:ss.sssZ (e.g., 2022-05-30T00:00:00.000Z)
- Short date: mm/dd/yyyy or dd/mm/yyyy (e.g., 04/24/2023 or 24/04/2023)
- Long date: MMMM dd, yyyy (e.g., April 24, 2023)
- RFC 2822: EEE, dd MMM yyyy HH:mm:ss GMT (e.g., Mon, 24 Apr 2023 00:00:00 GMT)
- Unix timestamp: The number of seconds or milliseconds since January 1, 1970 (e.g., 1640256000)
This tutorial will focus on the "dd/mm/yyyy" format, a standard format used in many countries. We'll explore various methods to parse, format, and manipulate dates in this format using JavaScript.
Parsing a Date
Parsing a date in JavaScript involves converting a string representation of a date into a Date
object. This is particularly useful when dealing with dates from external sources like APIs or user input fields.
JavaScript offers several methods for parsing dates, including Date.parse()
and the new Date()
constructor. It's important to note that the date string must be in a recognizable format for the parsing method to work. Common formats include ISO 8601, RFC 2822, and short date formats like "mm/dd/yyyy" or "dd/mm/yyyy."
Here are some examples:
// Parse an ISO date string
const ms = Date.parse("2022-05-30T00:00:00.000Z");
console.log(ms); // 1651296000000
// Parsing a non-ISO date string (may not work in some browsers)
const ms2 = Date.parse("30/05/2022");
console.log(ms2); // NaN
// Create a Date object from an ISO date string
const dt = new Date("2022-05-30T00:00:00.000Z");
console.log(dt); // 2022-05-30T00:00:00.000Z
// Create a Date object from numbers
const dt2 = new Date(2022, 4, 30); // Note: month is zero-based
console.log(dt2); // 2022-05-29T17:00:00.000Z
Consider exploring libraries mentioned later in the formatting section, as they provide robust parsing capabilities and support a wide range of date formats, including fuzzy parsing for interpreting partial or ambiguous dates.
Formatting a Date
There are various ways to format a date as "dd/mm/yyyy" in JavaScript, depending on your preferences and use case. Here are some possible solutions:
Using toLocaleDateString()
You can use the toLocaleDateString()
method with a locale parameter to get the date in "dd/mm/yyyy" format. For example:
const date = new Date();
// British English uses day-month-year order
console.log(date.toLocaleDateString('en-GB')); // 24/04/2023
// US English uses month-day-year order
console.log(date.toLocaleDateString("en-US")); // 04/24/2023
Keep in mind that toLocaleDateString()
relies on locale formatting rules and conventions and may not provide full control over the date string's format.
Manually Formatting
You can manually format the date by extracting the day, month, and year components and concatenating them with slashes. Don't forget to add leading zeros if necessary:
const today = new Date();
const yyyy = today.getFullYear();
let mm = today.getMonth() + 1; // month is zero-based
let dd = today.getDate();
if (dd < 10) dd = '0' + dd;
if (mm < 10) mm = '0' + mm;
const formatted = dd + '/' + mm + '/' + yyyy;
console.log(formatted); // 24/04/2023
Additionally, consider using libraries like Day.js, date-fns, or Luxon for more convenient date formatting and manipulation capabilities.
Using Libraries
Here are examples of how to format a date as "dd/mm/yyyy" using popular date manipulation libraries:
const dayjs = require("dayjs");
const dt = dayjs("2022-05-30T00:00:00.000Z");
const formatted = dt.format("DD/MM/YYYY");
console.log(formatted); // 30/05/2022
const { format } = require('date-fns');
const today = new Date();
const formatted = format(today, 'dd/MM/yyyy');
console.log(formatted); // 24/04/2023
const { DateTime } = require("luxon");
const dt = DateTime.fromFormat("31/12/2022", "dd/MM/yyyy");
const formatted = dt.toFormat("dd/MM/yyyy");
console.log(formatted); // 31/12/2022
These libraries offer powerful date manipulation capabilities and can simplify the process of working with dates in JavaScript. Choose the one that best fits your project's requirements and coding style.
Conclusion
In conclusion, formatting dates in JavaScript, especially in the "dd/mm/yyyy" format, can be accomplished using built-in methods or libraries, depending on your needs. By understanding the available options, you can ensure that your applications handle dates consistently and accurately.
Happy coding ?