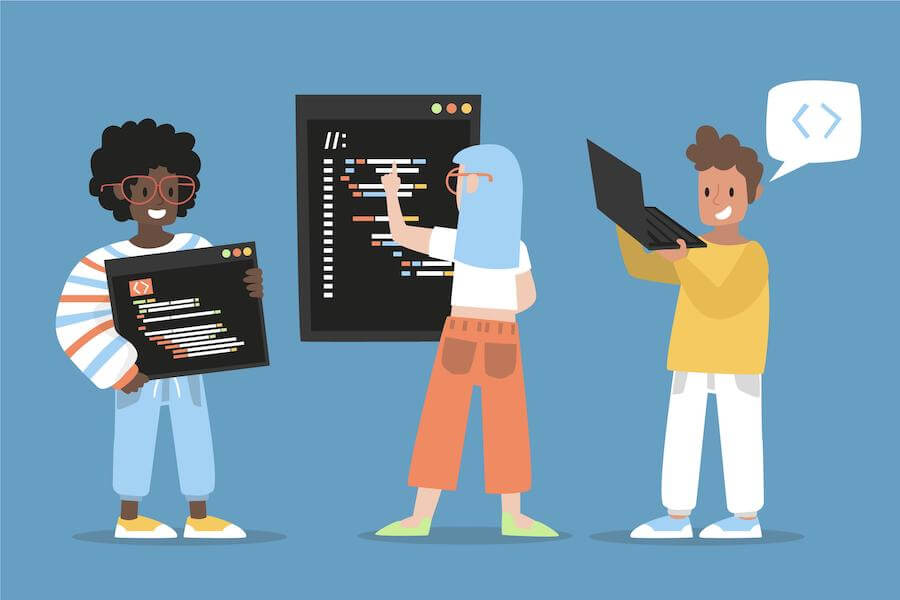
Here are 4 ways to combine strings in JavaScript. My favorite way is using Template Strings. Why? Because it’s more readable, no backslash to escape quotes, no awkward space separator, and no more messy plus operators
const name = 'Hoa Nguyen';
// Template Strings
`hi ${name}`;
// join() Method
['hi', name].join(' ');
// Concat() Method
''.concat('hi ', name);
// + Operator
'hi ' + name;
// RESULT
// hi Hoa Nguyen
1. Template Strings
If you come from another language, such as Ruby, you will be familiar with the term string interpolation. That's exactly what template strings are trying to achieve. It's a simple way to include expressions in your string creation which is readable and concise.
const name = 'Hoa Nguyen';
const country = '??';
Problem of Missing space in String concatenation
Before template strings, this would be the result of my string ?
"Hi, I'm " + name + "and I'm from " + country;
Did you catch my mistake? I'm missing a space. And that's a super common issue when concatenating strings.
// Hi, I'm Hoa Nguyenand I'm from ??
Resolved with Template Strings
With template strings, this is resolved. You write exactly how you want your string to appear. So it's very easy to spot if a space is missing. Super readable now ?
`Hi, I'm ${name} and I'm from ${country}`;
2. join()
The join
method combines the elements of an array and returns a string. Because it works
const array = ['My social links are: '];
const socialUsernames = ['Facebook: @xuho', 'Twitter: @nxhoase'].join(', '); // Facebook: @xuho, Twitter: @nxhoase
array.push(socialUsernames); // ['My social links are: ', 'Facebook: @xuho, Twitter: @nxhoase']
array.join(' ');
// My social links are: Facebook: @xuho, Twitter: @nxhoase
with an array, it's very handy if you want to add additional strings.
Customize Separator
The great thing about join
is that you can customize how your array elements get combined. You can do this by passing a separator in its parameter.
const array = ['My social links are: '];
const socialUsernames = ['Facebook: @xuho', 'Twitter: @nxhoase'].join(' - '); // Facebook: @xuho - Twitter: @nxhoase
array.push(socialUsernames);
array.join('');
// My social links are: Facebook: @xuho - Twitter: @nxhoase
3. concat()
With concat
, you can create a new string by calling the method on a string.
const instagram = '@xuho';
const twitter = '@nxhoase';
const tiktok = '@hoanx';
'My social links are: '.concat(instagram, ', ', twitter', ', tiktok);
// My social links are: @xuho, @nxhoase, @hoanx
Combining String with Array
You can also use concat
to combine a string with an array. When I pass an array argument, it will automatically convert the array items into a string separated by a comma ,
.
const array = ['xuho', 'nxhoase', 'hoanx'];
'My social links are: '.concat(array);
// My social links are: @xuho,@nxhoase,@hoanx
If you want it formatted better, we can use join
to customize our separator.
const array = ['xuho', 'nxhoase', 'hoanx'].join(', ');
'My social links are: '.concat(array);
// My social links are: @xuho, @nxhoase, @hoanx
4. Plus Operator (+)
One interesting thing about using the +
operator when combining strings. You can use to create a new string or you can mutate an existing string by appending to it.
Non-Mutative
Here we are using +
to create a brand-new string.
const instagram = '@xuho';
const twitter = '@nxhoase';
const tiktok = '@hoanx';
const newString = 'My social links are: ' + instagram + twitter + tiktok;
Mutative
We can also append it to an existing string using +=
. So if for some reason, you need a mutative approach, this might be an option for you.
let string = 'My social links are: ';
string += '@nxhoase' + 'hoanx';
// My social links are: @nxhoase@hoanx
Browser Support
Browser | Template String | join | concat | + |
---|---|---|---|---|
Internet Explorer | ❌ | ✅ IE 5.5 | ✅ IE 4 | ✅ IE 3 |
Edge | ✅ | ✅ | ✅ | ✅ |
Chrome | ✅ | ✅ | ✅ | ✅ |
Firefox | ✅ | ✅ | ✅ | ✅ |
Safari | ✅ | ✅ | ✅ | ✅ |
Performance Test
const icon = '?';
timeInLoop("template literal", 1e6, () => `hi ${icon}`);
timeInLoop("plus operator", 1e6, () => "hi " + icon);
timeInLoop("concat func", 1e6, () => ''.concat('hi ', icon));
timeInLoop("arr join", 1e6, () => ['hi', icon].join(' '));
/*
@perftests
run 1 million times each
template literal: 1e+6: 14.575ms
plus operator: 1e+6: 53.776ms
concat func: 1e+6: 391.723ms
arr join: 1e+6: 681.97ms
*/
Source: https://gist.github.com/funfunction/91b5876a5f562e1e352aed0fcabc3858