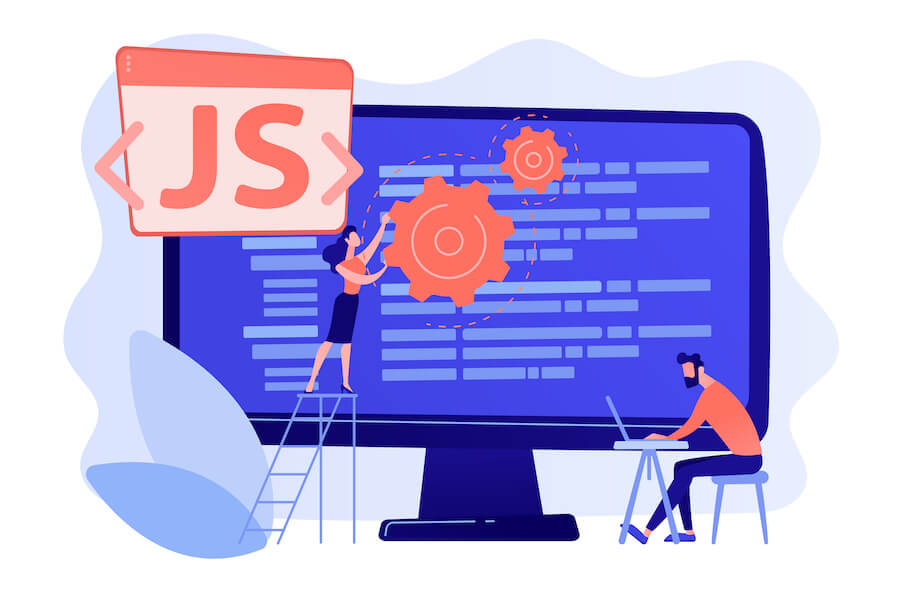
In this blog post, we will dive into the concepts of the global environment and the global object in JavaScript. Understanding these fundamental aspects will help us comprehend the execution context and the core components that the JavaScript engine provides when running our code. Let's explore the global execution context and its associated objects.
Global Execution Context
Introduction
When JavaScript code is executed, it operates within an execution context. This context acts as a wrapper around the code, providing an environment for the JavaScript engine to parse, verify, and execute it. The first execution context we encounter is the global execution context. Throughout this course, we will examine multiple execution contexts, but let's begin by focusing on the global execution context.
The Global Execution Context and Its Special Components
The global execution context is the primary execution context of a JavaScript program. It comes with a few special components that are automatically created for us. These components are the global object and a unique variable called 'this'.
The global execution context, often referred to as the global environment, encompasses code that is accessible and applicable everywhere within your program. It serves as the overarching context for your code. When we say "global," we mean that it is accessible from any part of your codebase.
The Global Object: A Collection of Name/Value Pairs
Within the global execution context, the JavaScript engine creates a Global Object for us. An object, in JavaScript, is simply a collection of name/value pairs. The Global Object contains various predefined properties and methods that we can utilize in our code. For instance, when running JavaScript in a browser, the Global Object is known as the "window" object. It represents the current browser window and provides access to browser-related functionalities.
Let's consider an example to better understand the Global Object. Suppose we define a variable named "name" and assign it the value "Nguyen Hoa" within the global execution context:
var name = "Nguyen Hoa";
In this case, the variable "name" becomes a property of the Global Object. We can access its value by referring to the Global Object directly:
console.log(window.name); // Output: Nguyen Hoa
The 'this' Variable: A Reference to the Global Object
Alongside the Global Object, the global execution context also creates a special variable called 'this.' This variable references the Global Object within the execution context. In the case of browser environments, such as Google Chrome or Firefox, the Global Object is the "window" object. Thus, when we refer to 'this' at the global level, it points to the same object as the "window" object.
Let's consider an example where we access the value of the 'this' variable at the global level (run in a browser environment):
console.log(this === window); // Output: true
In this case, the expression this === window
returns true because 'this' refers to the same object as the Global Object, which is the "window" object in a browser environment.
Exploring the Global Execution Context: An Empty JavaScript Program
Now, let's examine a practical example to observe the global execution context in action. Suppose we have an empty JavaScript file called "app.js." When this file is executed within a browser environment, an execution context is created. Despite the absence of code in the file, the JavaScript engine still generates the global execution context with the Global Object and the 'this' variable.
By accessing the browser's developer console and inspecting the execution context, we can observe that the Global Object is available as the "window" object. Additionally, the 'this' variable within the execution context also points to the "window" object. This showcases how the JavaScript engine automatically creates these components for us, even without any code.
Variables and Functions in the Global Execution Context
As we begin writing code within the global execution context, any variables or functions defined directly will be attached to the Global Object. This means that they become properties and methods of the Global Object, making them accessible globally throughout the codebase. For example, if we define a variable "a" with the value "Hello World!" and a function "b" within the global execution context, they become properties of the Global Object.
Conclusion
The global execution context plays a significant role in JavaScript, providing a foundation for code execution. It incorporates the global environment, comprising the Global Object and the 'this' variable. The Global Object acts as a collection of name/value pairs, allowing access to predefined properties and methods. The 'this' variable references the Global Object within the execution context. By understanding the global execution context and its associated components, we gain valuable insights into how JavaScript operates at the global level.